Android Static Analysis is a foundational approach to identifying vulnerabilities in applications without executing them. This blog provides insight into the tools and techniques required for effective analysis.
What is Android Static Analysis:
Android static analysis, analyzes an application’s codes, resources, and configuration files without executing the application, which is done with the help of some tools and a manual approach which we will discuss later.
Importance and need of static analysis:
Here we will discuss some key points that will make you clear about the importance and need for static analysis
- It helps in the identification of common vulnerabilities (SQL injection, hard-coded secrets, etc. in the early stages of development. Which indeed reduces costs, risk, and time.
- Static analysis checks code against standard and best practices. which includes naming conventions, formatting, and structure. Thus code review helps in overall code quality and prevents deviation from standard rules.
- Static analysis helps developers adhere to security standards like PCI DSS for payment security and HIPAA for protecting health information. It also helps create reports that can help in audits to ensure the app meets security standards.
- It aids in the identification of dependencies during the code change, which will indeed prevent new vulnerabilities and facilitate documentation.
- Tools used for static analysis help developers to improve their development skills.
Main focus areas in Android static analysis:
- Permissions: There are permissions in AndroidManifest.XML that can tell how an app intends to communicate with a device’s resources. Add check for Over-permission.
- Code and anti-reversing techniques: Some apps might be using obfuscation that shall be a signal of the need for deeper inspection.
- API Calls and Dependencies: Analysis can identify vulnerable API calls or outdated dependencies that may have known security risks.
- Data Storage: Examine how the app handles sensitive data or how the hardcoded credentials or unencrypted storage methods are in place. Proper handling of data is very important.
Tools used for static analysis:
Let us understand static analysis by doing it. For that, we should know the tools used for this purpose. So, here are some known and handy tools that we will discuss each tool one by one.
- APKTool: Used to unpack APK files and let you view and edit the AndroidManifest. xml and other resource files.
- MobSF (Mobile Security Framework): MobSF is a (Mobile Security Framework) that can be used as an all-in-one point for both static and dynamic analysis tools, useful for Android and iOS apps. It has very detailed reports and is relatively easy to use for beginners.
- Dex2Jar: Converts. Change. DEX→(dex files, compiled Android files) into. jar files making them readable by Java decompilers.
- JD-GUI: A Java Decompiler that helps you to see the source code of an app after you convert it. jar format.
- Androguard: A Python-based tool that can be used to analyze, decompile, and inspect Android apps bytecode.
- Ghidra: NSA developed a binary analysis suite for reverse engineering, this is a perfect tool for deep analysis of binaries and helps in advanced security inspection.
- APKleaks: An open-source tool for decompiling and making files readable. Though it relies on APKtool.
- Mobile Nuclei templates: Nuclei templates are available for the detection of vulnerabilities and misconfigurations.
Now, let’s have a look at each tool one by one. Let’s see how each tool gets installed, works, and helps us in Android static analysis.
APKTool:Â
Installation: APKtool installation involves two basic files APKTool .jar file and the installation script. which can be downloaded using these simple commands.
wget https://bitbucket.org/iBotPeaches/apktool/downloads/apktool_2.8.1.jar -O apktool.jar
wget https://raw.githubusercontent.com/iBotPeaches/Apktool/master/scripts/linux/apktool -O apktool
Next, move the files to the /usr/local/bin/ so that they can access system-wide. Now make the tool executable by giving it permissions by a simple command
sudo chmod +x /usr/local/bin/apktool
Usage of APKTool:
Once you have installed the tool. Now download an APK file to analyze. Here I have downloaded an open-source file InsecureBankv2.apk for analysis purposes. Now open the terminal and write apktool d <your-app.apk>
- For demonstration purposes we will be using: https://github.com/dineshshetty/Android-InsecureBankv2
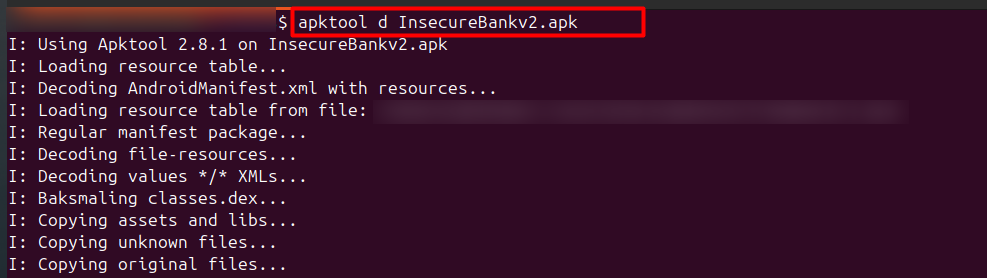
This command will create a folder with the same name as the APK you have and at the same location.

By navigating to the created folder you can find all the decompiled files for analysis. Now, navigate to each file created by the tool.
For example, by navigating the AndriodMenifest.xml
file you will find that there are lots of permissions, activities, and services that should be according to standards. In the given example there are permissions like USE_CREDENTIALS or READ_PROFILE
which indicate excess of permission.
This Android Manifest exports many components by declaring exported="true"
, thus making them accessible to other applications running on the device. Among these exported activities, the PostLogin, DoTransfer, ViewStatement, and ChangePassword could be invoked in several ways: Exposed activities include PostLogin, DoTransfer, ViewStatement, and ChangePassword, all of which can be called from other applications and may provide unauthorized access if proper security checks are not implemented.
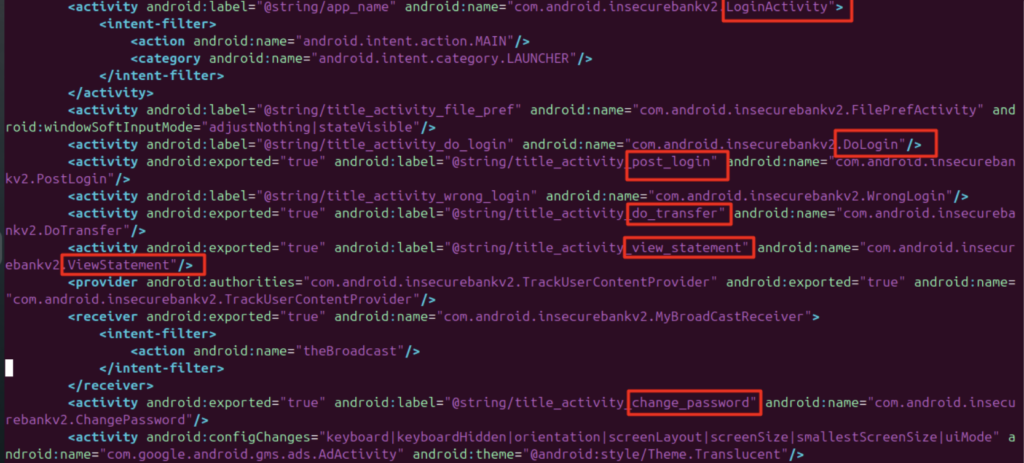
This manifest configures features of Google Play Services and Wallet API. Metadata google_play_services_version
checks the correctness of the version of Google Play Services for API compatibility. Setting wallet.api.enabled to true enables the functionality of Google Wallet for in-app payments. The EnableWalletOptimizationReceiver listens for optimization broadcasts, but it is set to android:exported=”false”; this increases security because access will be restricted to only the app itself.
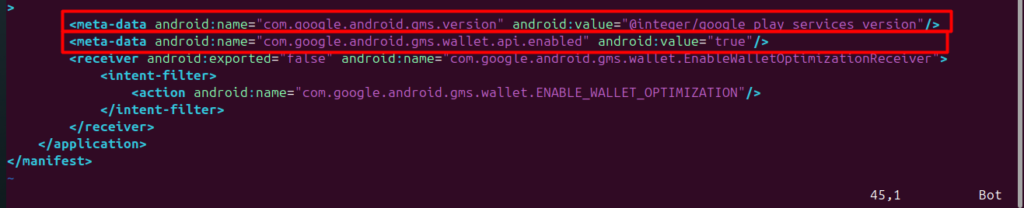
While doing static analysis you can also look for hardcoded credentials, unprotected APIS, insecure storage of sensitive data, weak cryptographic practices, vulnerabilities to injection attacks, certificate analyses, etc. Let’s do this with our next tool which is mobsf.
Mobsf (Mobile Security Framework):
It provides both static and dynamic analysis features, helping identify vulnerabilities, insecure configurations, and data privacy issues within APK, IPA, and ZIP files (source code). The best thing about mobsf is it provides a good graphical interface for analysis which makes it more user-friendly. Okay, let’s analyze our InsecureBankv2.APK file with mobsf. But before diving into analysis let’s talk about mobsf installation. You can install mobsf simply via docker using these two commands.
docker pull opensecurity/mobile-security-framework-mobsf
This will pull the repository and the next will run it on your local host.
docker run -it -p 8000:8000 opensecurity/mobile-security-framework-mobsf:latest
After running these commands the mobsf will be accessible at http://localhost:8000. Now that we are done with installation let’s start analysing the file. Once you start mobsf upload the file.
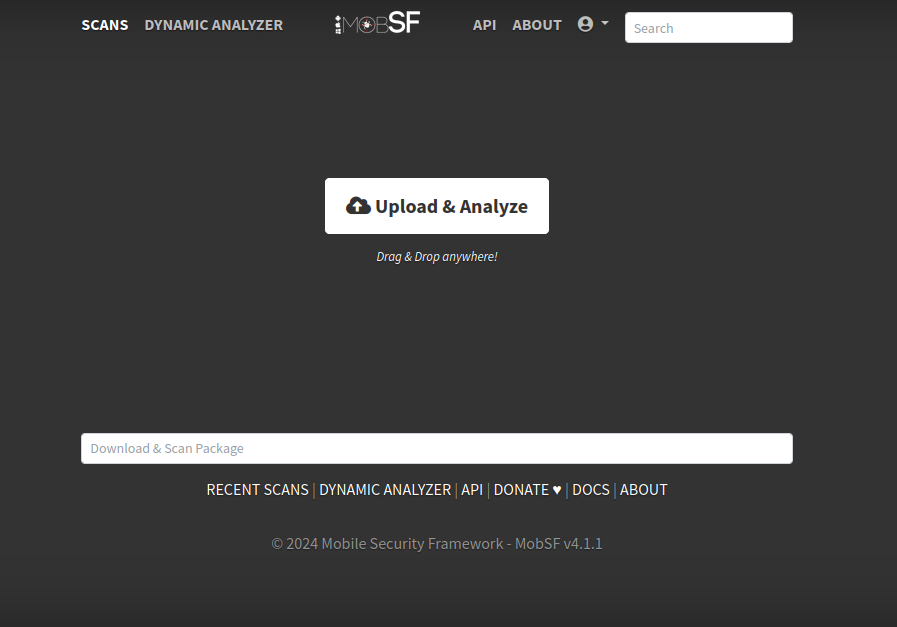
As I already have the InsecureBankv2.APK file I will upload it. Though you can upload zip, aab, .zip, .dex, and smali. As mobsf supports all these extensions. By clicking on the permission you can see the same permissions you have observed via APKtool. But in a graphical interface.
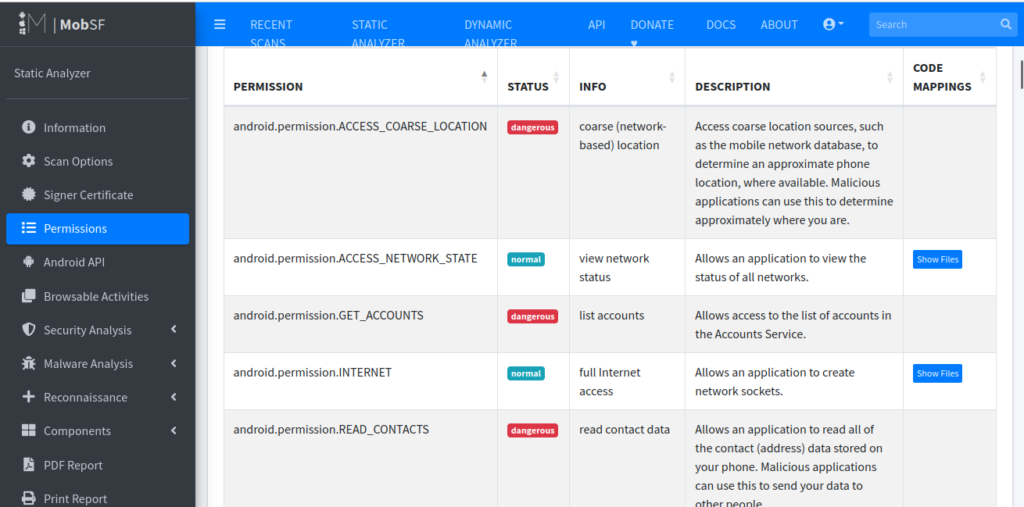
In the same way you can navigate to other components like activities to analyze them.
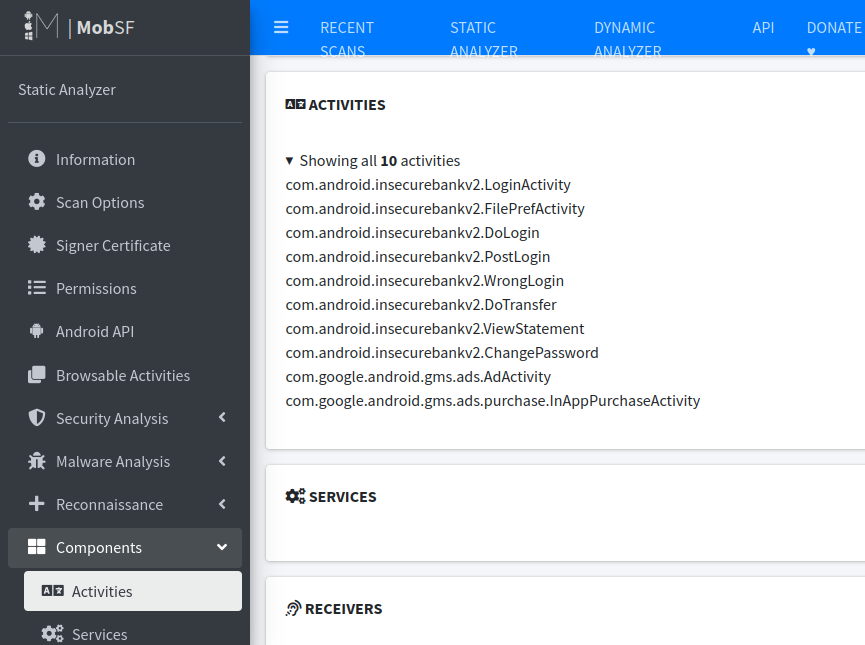
Okay now let’s see some things we have not seen with APKtool. For more clarity. Let’s see some hardcoded credentials and certificate analysis.
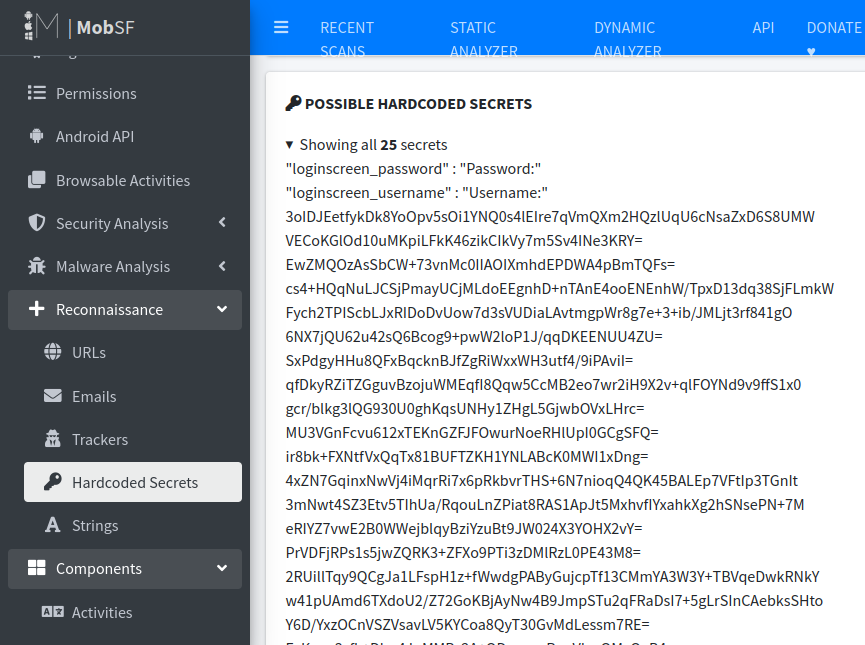
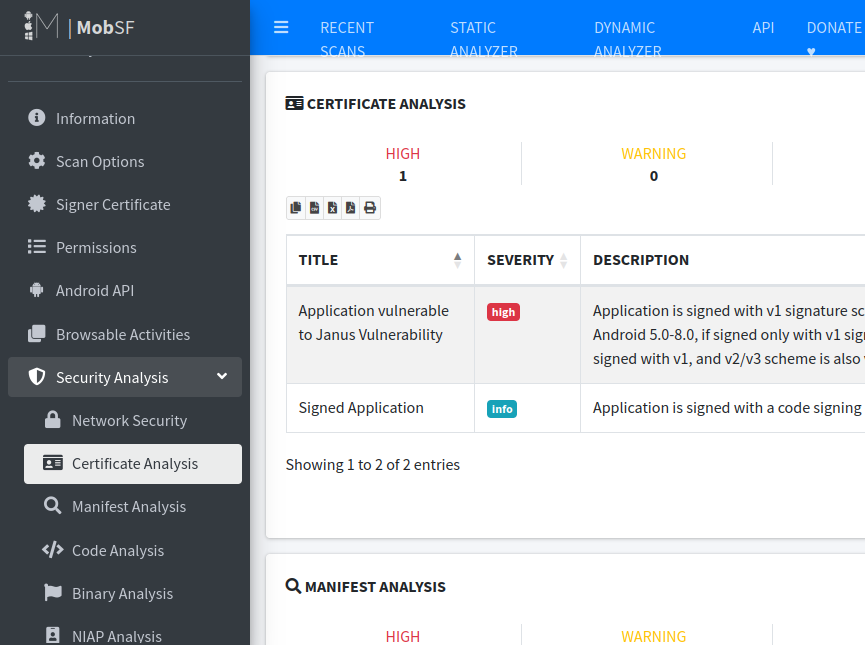
So, this is how you can easily navigate to each file component and perform static analysis with mobsf.
Dex2jar:
The dex2jar tool helps in converting Android app files (.dex files) into a .jar format, which later on can be decompiled to view the code. The speciality of this tool is as you know android apps are not distributed in a way that allows direct view of the source code. so this tool is helpful in code analysis for educational purposes, checking security aspects, and learning about how certain Android features are implemented.
Usage of dex2jar:
When it comes to usage you simply have to extract dex files from your apk file via the simple command
unzip sample.apk -d extracted_apk
Â
Now that once you have extracted the file classes.dex you can easily convert a .dex file to , a .jar for code analysis via a simple command.
./d2j-dex2jar.sh classes.dex
It will successfully, create a file named classes-dex2jar.jar in the same directory. Here comes another tool which is JD-GUI a java decompiler that helps you to see the source code once it’s converted to .jar.
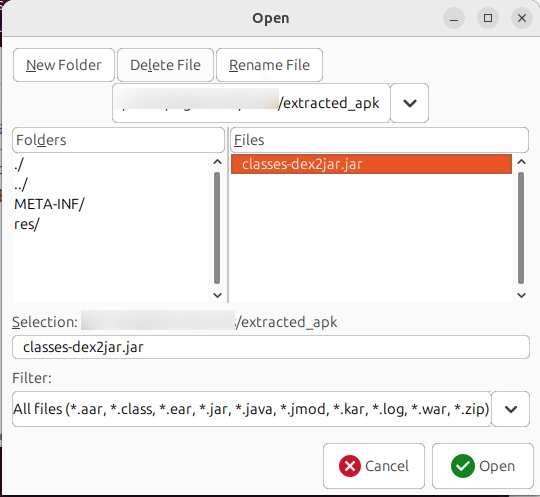
That’s how you can open a file in JD-GUI and analyze.
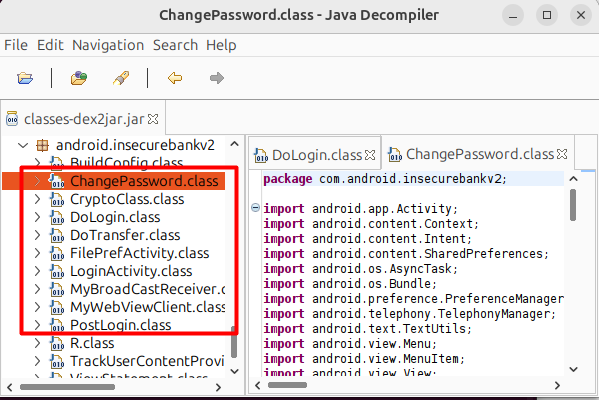
You must be wondering why JD-GUI is different from APKTool and Mobsf. It’s simply because JD-GUI focuses on Java code. Now how you can combine all and make your analysis better. it’s like if you have analyzed a file via APKtool or mobsf and you find a key you can now find this key in JD-GUI to find what that key is for, such as in API calls. In short, the JD-GUI helps in detecting what is the purpose of sensitive information.
One more thing that will help in analyzing source code in JD-GUI is its search feature which will help you locate where a is specific key, token, or secret is present. Download dex2jar:
Androgaud:
Androgaurd is another famous tool for android static analysis providing functionalities like decompiling, analyzing, and examining the structure of APK files and .dex files. You can insatall it:
Once installed, Androguard offers many commands and options for analyzing APK files. Here are some mentioned below.
androguard decompile -o output_folder app.apk
This will extract the APK’s contents into the output_folder for analysis. Though for viewing high-level details about APK files like permissions, activities, and services. Run the following command.
androguard axml app.apk
- To analyze a .dex file run
androguard decompile app.apk
- Androguaud can search for strings for that run androguard strings app.apk
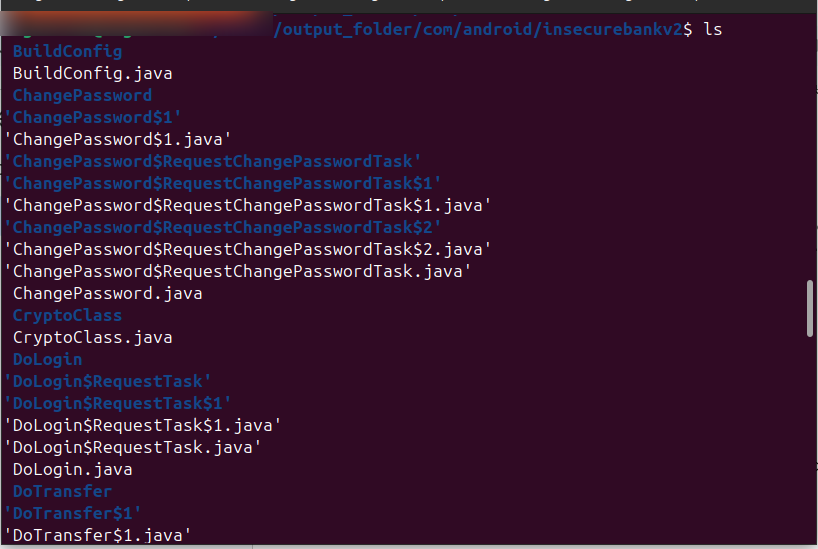
Ghidra:
Well this is another tool for static analysis which you can get by cloning or by downloading the latest release:
Once you have installed the tool move to the respective directory and run the command ./ghidraRun
Open ghidra and make a new project then import the file you want to analyze. Initial analysis with ghidra offers automatic analysis.
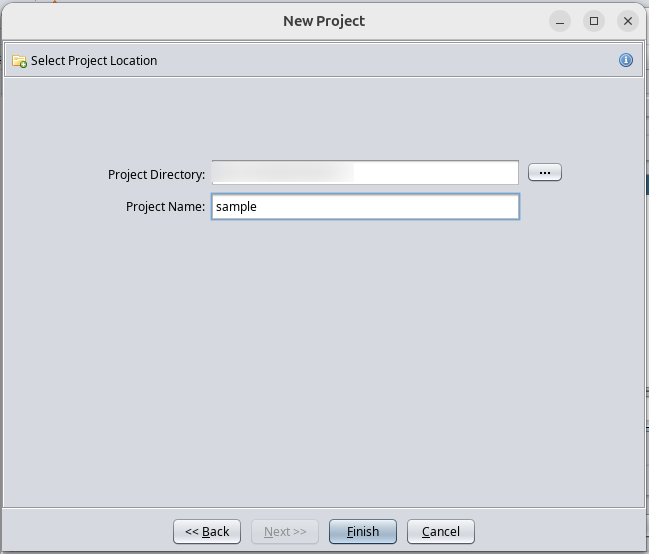
APKLeaks:
Another open-source tool available for static analysis is APKLeaks. However, it also relies on APKtool to decompile APK files into readable code and resources. For installation, you can clone the repository or you can pull it via docker.
$ git clone https://github.com/dwisiswant0/apkleaks
$ cd apkleaks/
$ pip3 install -r requirements.txt
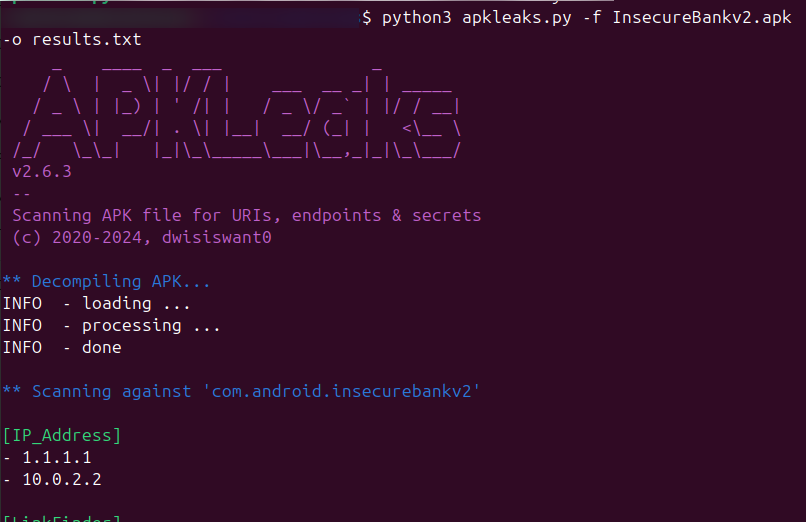
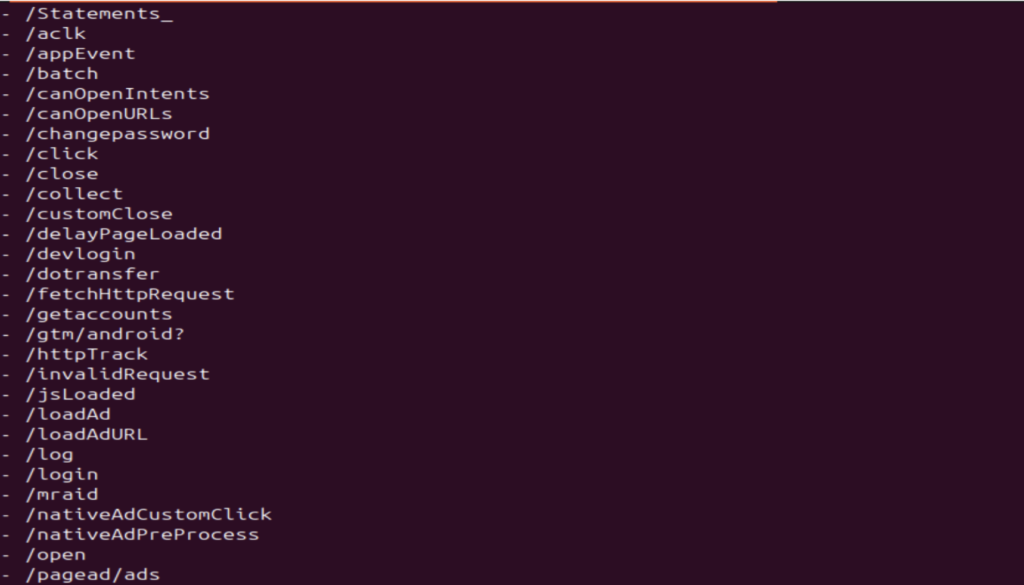
Here’s how it will decompile the file and make it readable.
From the output, we can see the apkleaks help in identifying domains, subdomains, API endpoints and some exposed keys by performing automated static code analysis which could really help in the OSINT process.
Well here’s one and last recommendation from my side, using Mobile-nuclei templates.Â
The main difference is nuclei are for network-based testing also they work by sending requests to URLs or IPs. It cannot read files directly. In nuclei templates, each template is a yaml file that defines a specific vulnerability or a misconfiguration. The templates can be clone via.
Conclusion:
Android Static Analysis is a great technique that helps identify security vulnerabilities in very early developmental processes so that the apps can be secured. Different tools such as apktool, MobSF, AndroGuard, dex2jar, JD-GUI, and Ghidra are used for decompiling APKs, inspecting code, reverse engineering, and identifying hardcoded secrets, each having their uniqueness. Together, these tools create a perfect approach to Android app security, for they help developers secure their users’ data by attempting to minimize security threats and create trust. Which is the main purpose of this.
So, Here we are ending this blog post with the hope that it might have helped you to understand Android static analysis. Happy Hacking 🙂
How can we help?
Finally, consider professional services from experts like Laburity. We specialize in static code analysis, supply chain security audits, and uncovering vulnerabilities, addressing hundreds of potential weaknesses for comprehensive security. You could schedule a call with us at https://calendly.com/laburity/meeting or reach out at [email protected]